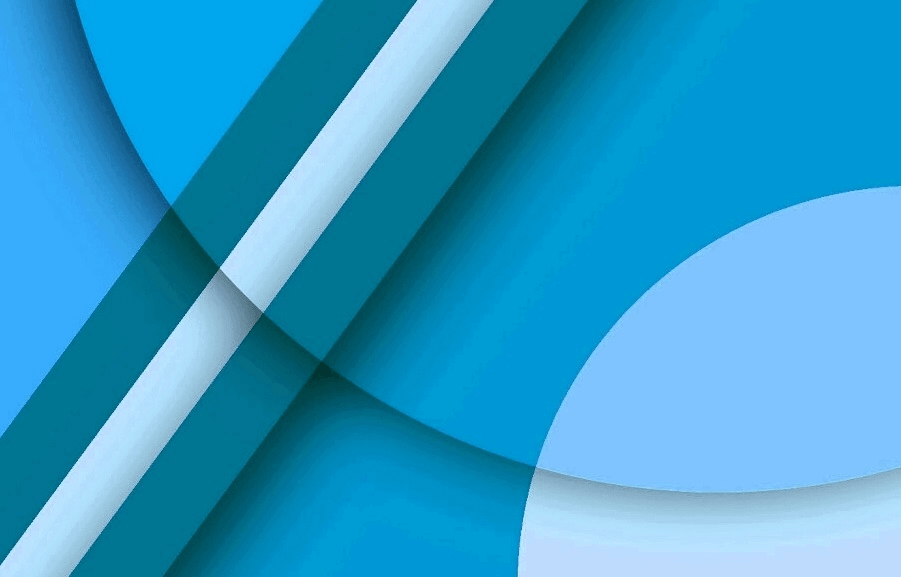
CSharp 去抖和节流
2021-04-20
去抖和节流,是前端频繁提及的概念。因为前端容易触及性能问题,比如窗口滑动、鼠标重复点击等等,如果没有去抖/节流/防重复提交这些操作,页面交互极有可能变得肉眼可见的卡顿。
现在 CSharp 编程中遇到类似情况,需要将同样的原理应用于后台编程。
一般情况下,我们可以使用第三方库,比如 Reactive Extension (类似于前端的 RxJS )。但是,我就只是要一个去抖和节流的功能,没必要引入一个第三方库吧。现实中项目如果要引入第三方库可能会引入各种问题。既然如此,何不重复造个轮子 😂
Talk is cheap, show me the code!
1 | /// <summary> |
- 节流很简单,就不过多解释了;
- 去抖,此处用到了三个关键函数: Task.Delay (类似于 js 中 setTimeout )、Task.ContinueWith (类似于 js 中的 Promise.then,不同的是后者接受两个参数,分别是成功回调和错误回调,而前者仅有一个回调,成功和错误都在一个里面)、CancellationTokenSource.Cancel。
参考链接
本文链接:
content_copy https://zxs66.github.io/2021/04/20/CSharp-debounce-and-throttle/